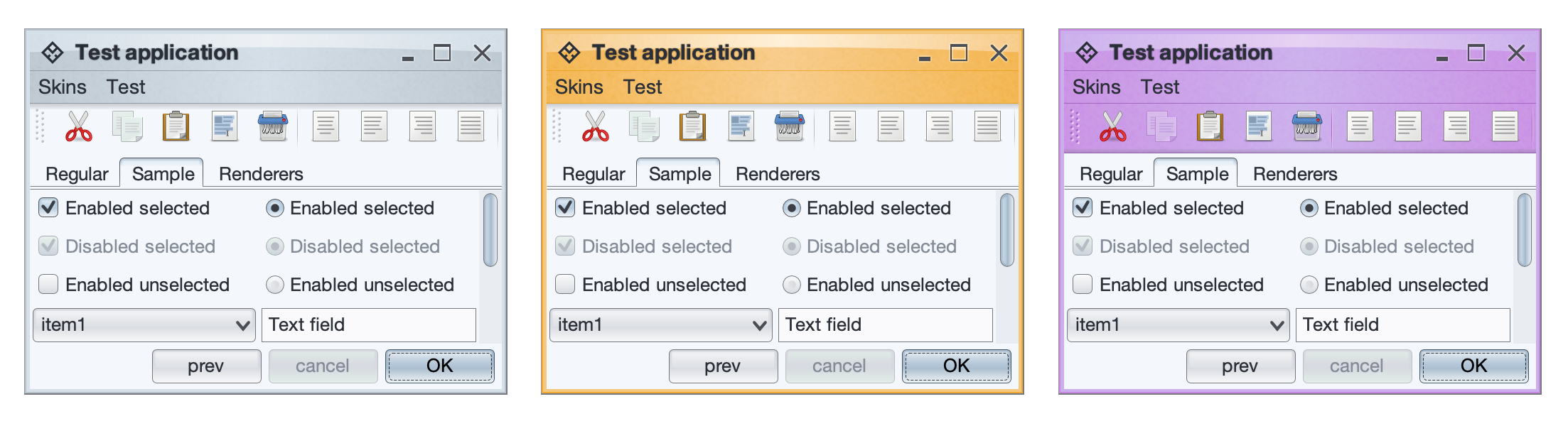
It gives me great pleasure to announce the fourth major release of Radiance. Let’s get to what’s been fixed, and what’s been added. First, I’m going to use emojis to mark different parts of it like this:
💔 marks an incompatible API / binary change
😻 marks new features
🤷♀️ marks bug fixes and general improvements
Dependencies for core libraries
- Gradle from
5.6.1
to 6.4.1
- Kotlin from
1.3.50
to 1.3.72
- Kotlin coroutines from
1.3.0
to 1.3.7
- Batik from
1.11
to 1.13
Substance
- 😻 A more flexible skin accent system
- 😻 New skins – Graphite Sienna, Sentinel and Harvest
- 😻 Support color references in color scheme files
- 😻 New Caps Lock indication on focused password fields
- 😻 New association kind for checkbox and radio button “boxes”
- 💔 Revisit APIs for loading color scheme bundles
- 💔 Remove the title pane heap status widget
- 🤷♀️ Use Helvetica Neue on macOS Catalina
- 🤷♀️ Visual refresh of checkbox marks
- 🤷♀️ Support for fallback fonts (CJK, etc)
- 🤷♀️ Fix for incorrect usage of
HIGHLIGHT_TEXT
association kind on renderers.
- 🤷♀️ Fix for background of popup menus opened from toolbar buttons.
- 🤷♀️ Fix improved contrast across core skins.
- 🤷♀️ Multiple fixes for table rollover hightlights and animations
- 🤷♀️ Fix for some components under very large font sizes
- 🤷♀️ Performance fix for column selection in large tables
- 🤷♀️ Fix for icons in file chooser drop downs
- 🤷♀️ Fix for incorrect bounds of maximized frames on secondary monitors
Flamingo
- 💔 General evolution of command button APIs
- 😻 Support for toggle split buttons
- 😻 Add API to wire notification on ribbon spinner changes
- 😻 Add API to wire notification on ribbon task selection
- 🤷♀️ Multiple focus traversal fixes for ribbon content
- 🤷♀️ Fixes for clipped wrapped ribbon components
- 🤷♀️ Fix to not use round corners on command buttons in menus
- 🤷♀️ Fix for crash in narrow command button panels
- 🤷♀️ Fix for crash in showing keytips on toggle anchored ribbon commands
- 🤷♀️ Fix for crash on showing keytips on undecorated windows
- 🤷♀️ Fix for dynamic changes to ribbon gallery content
- 🤷♀️ Fix for large icons on internal frames
- 🤷♀️ Use the public
Taskbar
API to set the ribbon frame dock icon
Trident
- 💔 Move all public APIs to
org.pushingpixels.trident.api
package
- 💔 Remove generic UI toolkit support and leave only Swing support
- 😻 Add support to provide dynamically computed
from
/ to
values on timelines.
Neon
- 💔 Move all public APIs to
org.pushingpixels.neon.api
package
Photon
Plasma
This release marks a special milestone for me. The very first Substance release happened exactly fifteen years ago, on May 30th 2005. I didn’t quite imagine that I’d be here today, still working on the same codebase.
There’s still a long road ahead to continue exploring the never-ending depths of what it takes to write elegant and high-performing desktop applications in Swing. If you’re in the business of writing just such apps, I’d love for you to take this Radiance release for a spin. Click here to get the instructions on how to add Radiance to your builds. And don’t forget that all of the modules require Java 9 to build and run.
Here’s to the next fifteen years!
Radiance comes with a number of sample / demo apps that showcase the flexibility and power of its APIs. One of those demos is Lumen. Its main goal is to highlight the feature set of the Trident animation library. Lumen uses MusicBrainz JSON web service to search for all albums of the specific artist, and for the list of tracks on individual albums. Sending requests and parsing responses is done with Retrofit and Moshi. Lucent is the port of Lumen to Kotlin.
Let’s see how it works together in Kotlin.
We start by adding the build dependencies on Retrofit and Moshi:
dependencies {
implementation "com.squareup.retrofit2:retrofit:2.9.0"
implementation "com.squareup.retrofit2:converter-moshi:2.9.0"
}
Next, we define our service interface that maps to MusicBrainz APIs:
private interface MusicBrainzService {
@GET("/ws/2/release?type=album&fmt=json")
fun getReleases(@Query("artist") artistId: String): Call<ReleaseList>
@GET("/ws/2/release/{release}?inc=recordings&fmt=json")
fun getRelease(@Path("release") releaseId: String): Call<Release>
companion object {
const val API_URL = "https://musicbrainz.org/"
}
}
Note the usage of fmt=json
attribute in all @GET
functions, and usage of @Query
and @Path
that matches the expected endpoint contracts.
The data classes map to the matching MusicBrainz entities, using @field:Json
annotation with the matching name
attribute, along with @Json
annotation on one of the data classes to properly map it to the matching JSON tags:
data class SearchResultRelease(
@field:Json(name = "id") val id: String?,
@field:Json(name = "title") val title: String?,
@field:Json(name = "artist") var artist: String?,
@field:Json(name = "date") val date: String?,
@field:Json(name = "release-events") val releaseEvents: List<ReleaseEvent>,
@field:Json(name = "asin") val asin: String?)
data class Area(
@field:Json(name = "disambiguation") val disambiguation: String?,
@field:Json(name = "id") val id: String?,
@field:Json(name = "name") var name: String?,
@field:Json(name = "sort-name") val sortName: String?,
@field:Json(name = "iso-3166-1-codes") val iso31661Codes: List<String>)
data class Medium(
@field:Json(name = "tracks") val tracks: List<Track>)
data class Release(
@field:Json(name = "id") val id: String?,
@field:Json(name = "title") val title: String?,
@field:Json(name = "date") val date: String?,
@field:Json(name = "media") val media: List<Medium>,
@field:Json(name = "asin") val asin: String?)
data class ReleaseEvent(
@field:Json(name = "date") val date: String?,
@field:Json(name = "area") val area: Area?)
@Json(name = "release-list")
data class ReleaseList(
@field:Json(name = "count") val count: Int?,
@field:Json(name = "releases") val releases: List<SearchResultRelease>)
data class Track(
@field:Json(name = "title") val title: String?,
@field:Json(name = "length") val length: Int?)
Now we can create a Retrofit
object and fire off our request:
val retrofit = Retrofit.Builder()
.baseUrl(MusicBrainzService.API_URL)
.client(getHttpClient())
.addConverterFactory(MoshiConverterFactory.create())
.build()
val service = retrofit.create(MusicBrainzService::class.java)
val releaseResponse = service.getReleases(artistId).execute()
val releases = releaseResponse.body()
And to get the list of tracks for the specific album:
fun doTrackSearch(releaseId: String): List<Track> {
val retrofit = Retrofit.Builder()
.baseUrl(MusicBrainzService.API_URL)
.client(getHttpClient())
.addConverterFactory(MoshiConverterFactory.create())
.build()
val service = retrofit.create(MusicBrainzService::class.java)
val releaseResponse = service.getRelease(releaseId).execute()
val release = releaseResponse.body()
return release!!.media[0].tracks
}
Where the OkHttpClient
is configured like this:
private fun getHttpClient(): OkHttpClient {
val okHttpBuilder = OkHttpClient.Builder()
okHttpBuilder.addInterceptor { chain ->
val requestWithUserAgent = chain.request().newBuilder()
.header("User-Agent", "My custom user agent")
.build()
chain.proceed(requestWithUserAgent)
}
return okHttpBuilder.build()
}
This is it. No messy handling of HTTP requests, no manual parsing of JSON responses. All driven by metadata and encapsulated by Kotlin data classes.
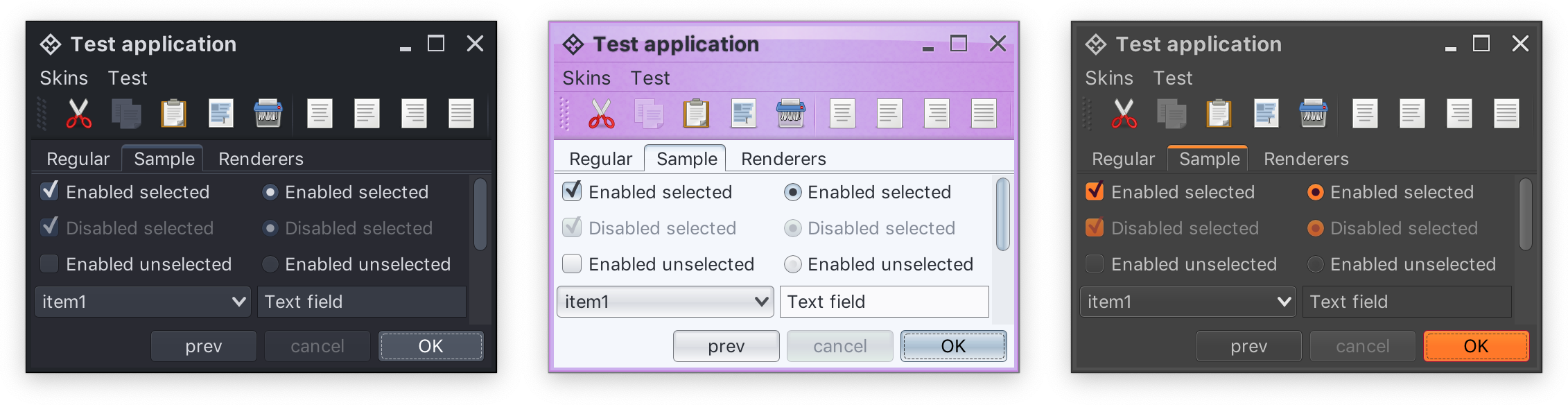
It gives me great pleasure to announce the third major release of Radiance. Let’s get to what’s been fixed, and what’s been added. First, I’m going to use emojis to mark different parts of it like this:
💔 marks an incompatible API / binary change
😻 marks new features
🤷♀️ marks bug fixes and general improvements
Substance
- 😻 New skins – Nebula Amethyst, Night Shade and Graphite Sunset
- 🤷♀️ Fix for disappearing internal frame title pane buttons
- 🤷♀️ Fix for crash during initialization
- 🤷♀️ Fix for
OutOfMemoryError
on sliders with large model ranges
- 🤷♀️ Fix for slider tracks under dark skins
- 💔 Fix for incorrect tracking of state-based alpha values in color scheme bundles
- 🤷♀️ Fix for drop shadows under some skins
- 🤷♀️ Fix for contrast ratio of highlighted content under Sahara skin
- 🤷♀️ Fix for antialiased rendering of pasted text content
Flamingo
Trident
Photon
The first Radiance release focused on bringing all the different Swing open-source projects that I’ve been working on since 2005 under one roof. The second Radiance release was about making them work much better together. And this one (code-named Coral) is about covering major functionality gaps that were missing up until now.
There’s still a long road ahead to continue exploring the never-ending depths of what it takes to write elegant and high-performing desktop applications in Swing. If you’re in the business of writing just such apps, I’d love for you to take this third Radiance release for a spin. Click here to get the instructions on how to add Radiance to your Gradle / Maven / Ivy / Leiningen / Bazel builds. And don’t forget that all of the modules require Java 9 to build and run.
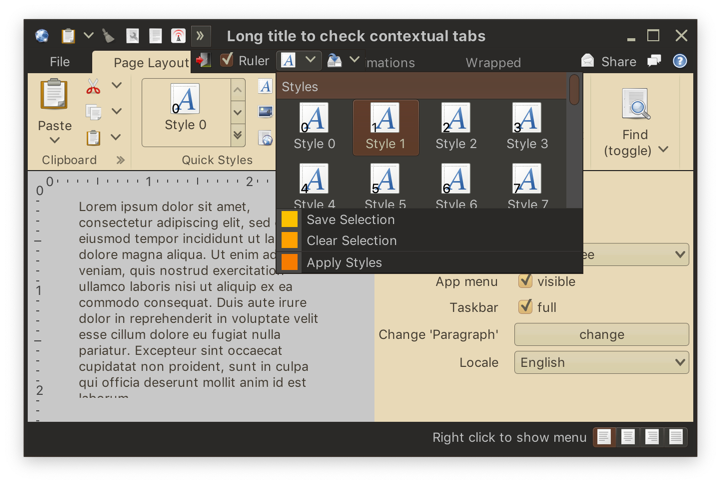
It gives me great pleasure to announce the second major release of Radiance. It was all ready to go as 2.0.0, but what’s a release really if a blocker bug doesn’t make it in? So instead, you get to get 2.0.1 for now – pending any other blockers that would require a couple more minor re-spins. Anyway, let’s get to what’s new. First, I’m going to use emojis to mark different parts of it like this:
💔 marks an incompatible API / binary change
😻 marks new features
🤷♀️ marks bug fixes and general improvements
General
- 💔 Java 9 is the new minimum requirement for build time and runtime of all Radiance modules
Modules
- 💔 Removed Spoonbill (SVNKit-powered implementation of Flamingo’s breadcrumb bar
- 😻 Added Meteor – Kotlin extensions for core Swing APIs
- 😻 Added Ember – Kotlin extensions for
SubstanceCortex
APIs
- 🤷♀️ Renamed Kormorant to Plasma
- 🤷♀️ All core Kotlin modules (Ember, Meteor, Plasma) moved under the top-level
kotlin-ext
folder
- 🤷♀️ Jitterbug (visual tool for editing Substance color schemes) renamed to Apollo
- 😻 Added Ion – sample walkthroughs for replacing
SwingWorker
with Kotlin coroutines
Neon
- 💔 An almost complete rewrite of
NeonIcon
APIs
- 💔 Most Flamingo and Substance APIs moved off of
ResizableIcon
and to ResizableIcon.Factory
- 💔 Moved some icon colorization APIs from Substance to Neon
- 💔 Removed usage of
UITable
from FontPolicy
API
Photon
- 💔 Removed default public no-argument constructor from bundled templates for Java and Kotlin targets
Trident
- 💔 Moved to builder-based construction of timelines
Substance
- 😻 New
Graphite Electric
skin
- 😻 New APIs for working with complex renderers, including built-in animations
- 🤷♀️ Fix for incorrect offsets of rotated texts
- 🤷♀️ Fix for inconsistent font metrics between preferred size and rendering passes
- 🤷♀️ Fix for incorrect vertical position of icons in
JOptionPane
- 🤷♀️ Fix for crash in showing
JColorChooser
dialog
- 💔 Moved all three Office 2007 skins to the extras pack
Flamingo
- 💔 Moved all lower-level components (command button, command button strip. command popup menu, command button panel) to the new world based on content models, presentation models and projections
- 😻 Added support for placing any ribbon content (including components, application menu links and galleries) in the taskbar
- 😻 Added support for taskbar overflow (including built-in horizontal scrolling)
- 💔 Keytips for taskbar content are controlled by keytip policy
- 😻 Added support for separate keytips on action and secondary / popup areas of command buttons
- 😻 Added support for global contextual menu on the ribbon
- 🤷♀️ Added complete documentation
The first Radiance release focused on bringing all the different Swing open-source projects that I’ve been working on since 2005 under one roof. This release (code-named Beryl) is about making them work much better together. And it’s also about making it just a bit easier to use Flamingo components in general, and the ribbon in particular, in what one might call serious, if not even boring, business applications.
There’s still a long road ahead to continue exploring the never-ending depths of what it takes to write elegant and high-performing desktop applications in Swing. If you’re in the business of writing just such apps, I’d love for you to take this second Radiance release for a spin. Click here to get the instructions on how to add Radiance to your Gradle / Maven / Ivy / Leiningen / Bazel builds. And don’t forget that all of the modules require Java 9 to build and run.