Radiance comes with a number of sample / demo apps that showcase the flexibility and power of its APIs. One of those demos is Lumen. Its main goal is to highlight the feature set of the Trident animation library. Lumen uses MusicBrainz JSON web service to search for all albums of the specific artist, and for the list of tracks on individual albums. Sending requests and parsing responses is done with Retrofit and Moshi. Lucent is the port of Lumen to Kotlin.
Let’s see how it works together in Kotlin.
We start by adding the build dependencies on Retrofit and Moshi:
dependencies {
implementation "com.squareup.retrofit2:retrofit:2.9.0"
implementation "com.squareup.retrofit2:converter-moshi:2.9.0"
}
Next, we define our service interface that maps to MusicBrainz APIs:
private interface MusicBrainzService {
@GET("/ws/2/release?type=album&fmt=json")
fun getReleases(@Query("artist") artistId: String): Call<ReleaseList>
@GET("/ws/2/release/{release}?inc=recordings&fmt=json")
fun getRelease(@Path("release") releaseId: String): Call<Release>
companion object {
const val API_URL = "https://musicbrainz.org/"
}
}
Note the usage of fmt=json
attribute in all @GET
functions, and usage of @Query
and @Path
that matches the expected endpoint contracts.
The data classes map to the matching MusicBrainz entities, using @field:Json
annotation with the matching name
attribute, along with @Json
annotation on one of the data classes to properly map it to the matching JSON tags:
data class SearchResultRelease(
@field:Json(name = "id") val id: String?,
@field:Json(name = "title") val title: String?,
@field:Json(name = "artist") var artist: String?,
@field:Json(name = "date") val date: String?,
@field:Json(name = "release-events") val releaseEvents: List<ReleaseEvent>,
@field:Json(name = "asin") val asin: String?)
data class Area(
@field:Json(name = "disambiguation") val disambiguation: String?,
@field:Json(name = "id") val id: String?,
@field:Json(name = "name") var name: String?,
@field:Json(name = "sort-name") val sortName: String?,
@field:Json(name = "iso-3166-1-codes") val iso31661Codes: List<String>)
data class Medium(
@field:Json(name = "tracks") val tracks: List<Track>)
data class Release(
@field:Json(name = "id") val id: String?,
@field:Json(name = "title") val title: String?,
@field:Json(name = "date") val date: String?,
@field:Json(name = "media") val media: List<Medium>,
@field:Json(name = "asin") val asin: String?)
data class ReleaseEvent(
@field:Json(name = "date") val date: String?,
@field:Json(name = "area") val area: Area?)
@Json(name = "release-list")
data class ReleaseList(
@field:Json(name = "count") val count: Int?,
@field:Json(name = "releases") val releases: List<SearchResultRelease>)
data class Track(
@field:Json(name = "title") val title: String?,
@field:Json(name = "length") val length: Int?)
Now we can create a Retrofit
object and fire off our request:
val retrofit = Retrofit.Builder()
.baseUrl(MusicBrainzService.API_URL)
.client(getHttpClient())
.addConverterFactory(MoshiConverterFactory.create())
.build()
val service = retrofit.create(MusicBrainzService::class.java)
val releaseResponse = service.getReleases(artistId).execute()
val releases = releaseResponse.body()
And to get the list of tracks for the specific album:
fun doTrackSearch(releaseId: String): List<Track> {
val retrofit = Retrofit.Builder()
.baseUrl(MusicBrainzService.API_URL)
.client(getHttpClient())
.addConverterFactory(MoshiConverterFactory.create())
.build()
val service = retrofit.create(MusicBrainzService::class.java)
val releaseResponse = service.getRelease(releaseId).execute()
val release = releaseResponse.body()
return release!!.media[0].tracks
}
Where the OkHttpClient
is configured like this:
private fun getHttpClient(): OkHttpClient {
val okHttpBuilder = OkHttpClient.Builder()
okHttpBuilder.addInterceptor { chain ->
val requestWithUserAgent = chain.request().newBuilder()
.header("User-Agent", "My custom user agent")
.build()
chain.proceed(requestWithUserAgent)
}
return okHttpBuilder.build()
}
This is it. No messy handling of HTTP requests, no manual parsing of JSON responses. All driven by metadata and encapsulated by Kotlin data classes.
Continuing the ongoing series of interviews with creative artists working on various aspects of movie and TV productions, I’m delighted to welcome Frank Walsh. In this interview he talks about the beginning of his career, how technology continues to reshape the industry, finding the next challenge and never stopping to learn new things, and the complexity of the art and craft of production design. Around these topics and more, Frank dives deep into his work on the fabulously designed first season of “Carnival Row”.
Kirill: Please tell us about yourself and the path that took you to where you are today.
Frank: I took a Three Dimensional Design course at Hornsey College of Art for my BA, and it was a fairly unique course in design at that time. Students were designing furniture, architecture, ceramics, silversmithing – and that’s where I started. It was during that course I met a tutor there who made me consider that silversmithing was just one manifestation of a design process. I got interested in designing furniture, this expanded into the interiors for my furniture and finally architecture, and ultimately that got me into the prestige London centre of creative arts, The Royal College of Art, to do my master’s degree in architectural design. Whilst it was there that my deeper interest in film and television came about when I took a Liberal Studies module and Christopher Frayling tutored me on Sergio Leone and spaghetti westerns.
That was the first time I’d ever sat down and been introduced to the idea of analysing a movie scene by scene, and understanding how the elements of a movie go together – from the sets, the cinematography, costume, sound, and music. It opened a whole new world to me, and I just thought the complexity was so fascinating. So in the weeks running up to my graduation, I started to write a lot of letters to anybody I could find in the industry.
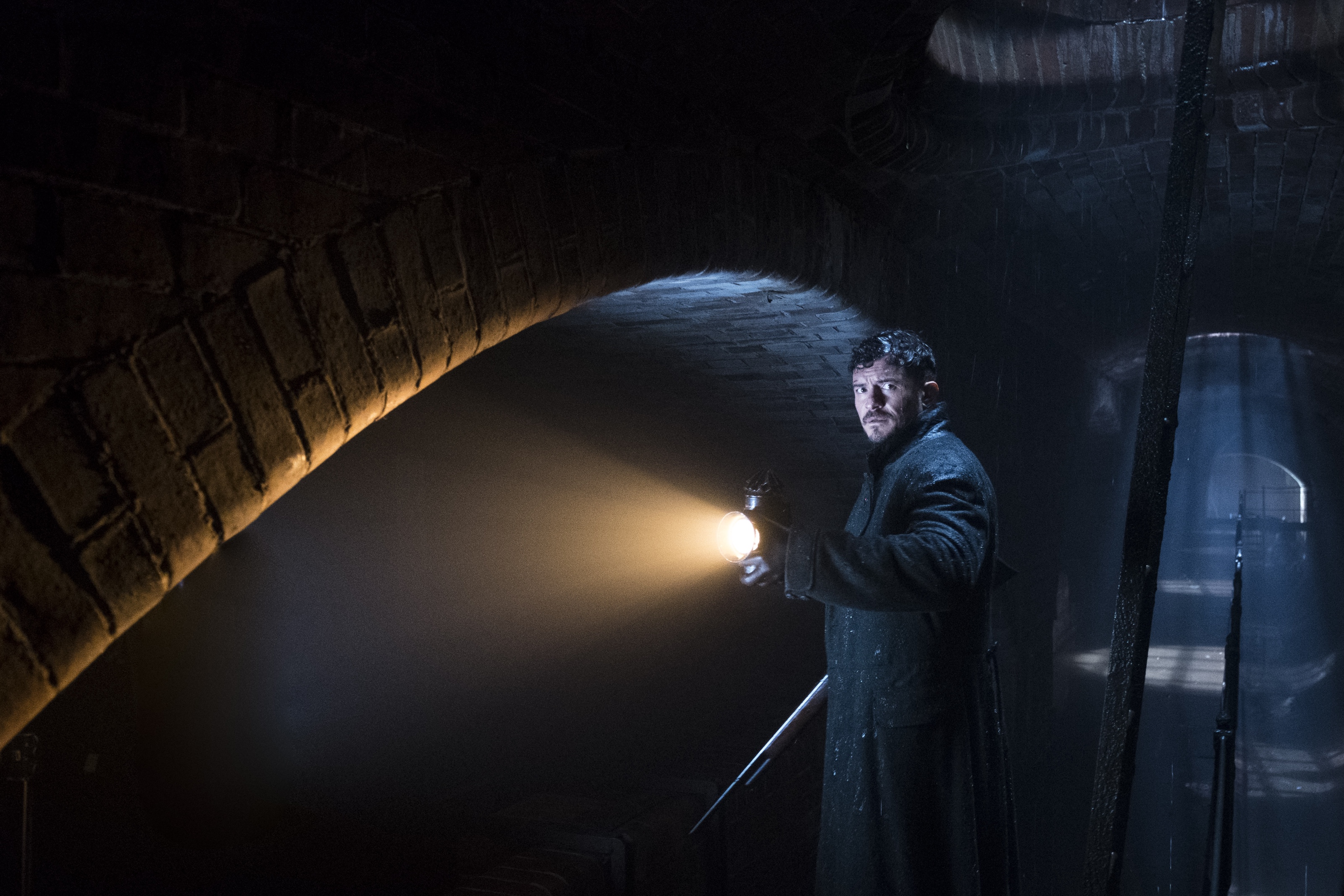
On the sets of “Carnival Row”. Production design by Frank Walsh. Courtesy of Amazon Studios.
Through one letter, I was introduced to the art department on the Bond movie “Moonraker”. Ken Adam was designing it, and it was one of those lucky moments that shape a life. One of the juniors was leaving to go onto another film, and I was just there at the right moment and coming from a post-graduate university education into the industry, it was quite an unusual route at that time to get into the art department on a movie.
It was a great privilege having Ken Adam as my first boss. I used to look after his office, sort out all his drawings and whatever else there was to do to assist. The department at Pinewood was charged mainly with shooting all the visual effects and miniatures that appear in the film, so I was immersed in that very special aspect of film making from the very start. Working and learning from Ernie Archer who had won an Oscar for “Nicholas and Alexander” and a nomination for designing “2001: A Space Odyssey” the assistant art director on it and Peter Lamont who later gained an Oscar for his designs for “Titanic” who was the visual effects art director. The Director being Derek Meddings who during filming was awarded an Oscar for his pioneering effects work on Superman, and subsequently was again nominated for ‘Moonraker’. So I enjoyed a fantastic grounding early on in my career with that film and saw how solutions can often be found through ingenuity rather than always technology.
Then I had a fortunate period of working on several films with Elliott Scott whose background was from the very early post-war years through films such as the Indiana Jones films, “Labyrinth” and “Who Framed Roger Rabbit”, and with three Oscar nominations to his credit. It gave me grounding in the depth of the art department for film, what sets are about and how you integrate with all the other departments. It was all about being aware that the art department is almost like a catalyst to the production, a place from which all the information comes from. It involves all the other areas, including special effects and visual effects.
This was the early days of ILM, and on one film I was working with Dennis Muren. Pre computers, the art department were hand-drawing all the camera projections for the VFX elements. I learned how to use the American Cinematographer Manual to work out camera angles, lenses, depth of field. Those were the days when you’ve plotted everything in the art department for visual effects. It taught me that the visual effects and the art department are basically one entity and hopefully going forward in the future we meld into one department.
I was very fortunate to have had an all-encompassing training very early on, starting from making tea to standing by, storyboarding, drafting sets, graphics, set dressing and designing.
Kirill: If I look back at the last 10 years or so, the presence of the smartphone technology in my life has changed so much of my everyday routine, and yet almost all of those changes did not seem to be major when each one of them was introduced. If you look at the last 30 years of technology in visual storytelling and how it changed the art department, what do you see?
Frank: It certainly has evolved. I recall trying to hide a non-period telegraph pole with a tree, using signalling flags as it was before mobile phones. I’ve been very lucky in that things have happened to me at just the right moments in the industry. When I started, we didn’t have any computers. You did budgets by hand on a calculator, and it was laid out as a paper spreadsheet. Doing scheduling was all done by hand. As computers came out, I was just in the right place at the right time and was willing to understand and absorb new technology even as many of my superiors rejected its implementation.
Working on “The Hitchhiker’s Guide to the Galaxy” with Joel Collins with whom you spoke a couple of years ago. It was one of his first productions, and both he and Dan May had come from a background where there were comfortable with emerging technology. For them, the challenge probably was moving into the bigger feature film world, and my competency to guide them as a supervising art director and assist them with the process of delivering the sets was a great collaboration. For I was learning from them as much as they from me, about how the technology was changing, and that’s something I’ve always tried to do. My mantra is that you should never stop learning. And embracing developing technology and ensuring it effectively used.
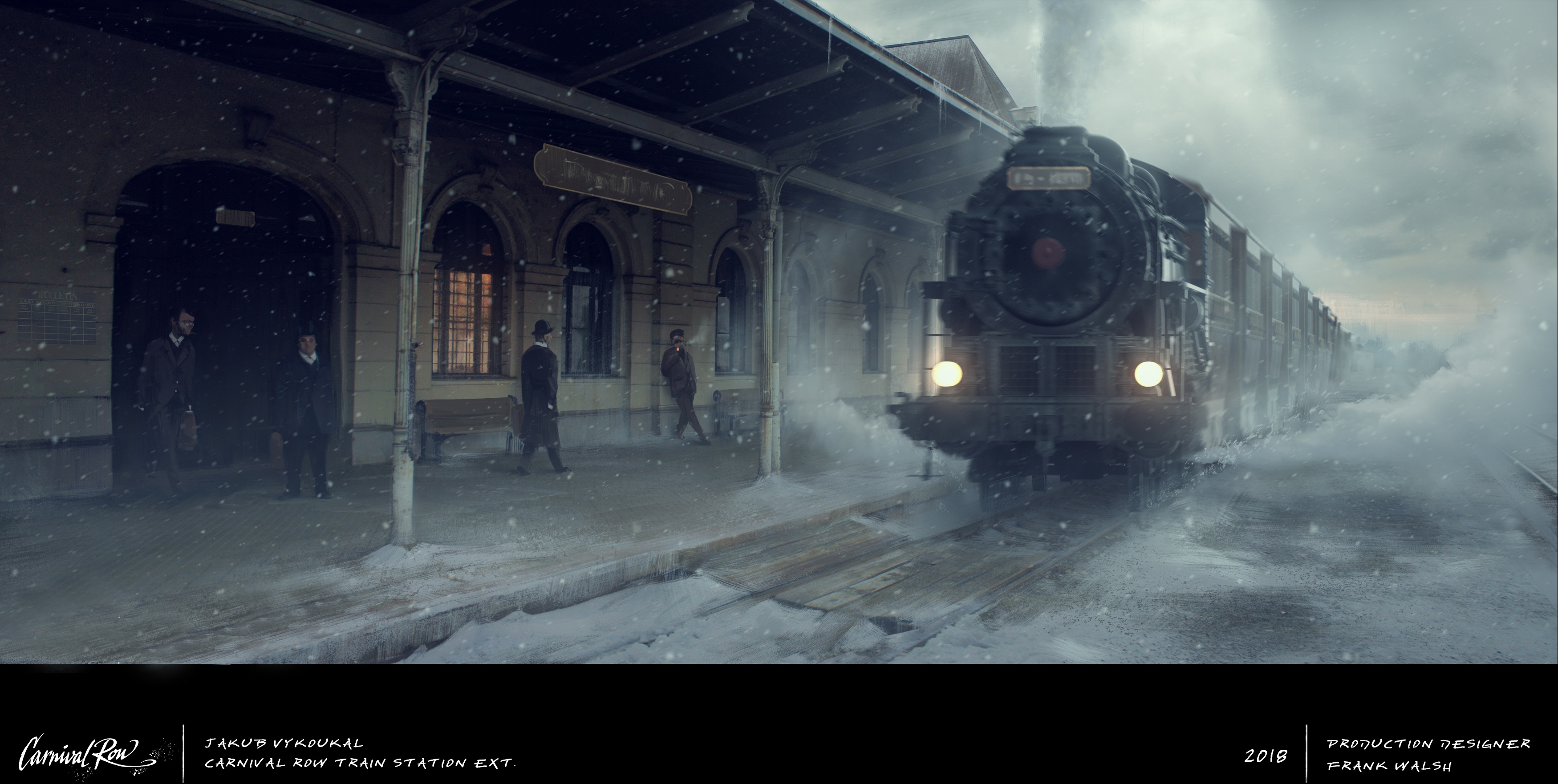
Concept art for “Carnival Row”, train station exterior. Production design by Frank Walsh. Courtesy of Frank Walsh and Amazon Studios.
However, when I teach, I do still try and encourage my students to get themselves grounded in the old approaches – technical drawing with a pencil. That mental process is critical. The amount of effort you do to do a paper drawing is different from what you do on a computer. It makes you much more focused on the process and being economical. It’s just physically tiring to cover a piece of paper with a lot of drawings. I think it gets them to an understanding that you have to analyse things. The new technology makes things happen very quickly and very rapidly. But there are certain cases where even though you have that technology, sometimes it’s better not to use it or rely on it alone.
Sometimes I feel there’s an overuse of visual effects that is detrimental to the storytelling. I’m not decrying anything in particular, and there are great movies that are very born out of visual effects. But there is a need to be respectful that the story comes first. My sets are always designed around making an environment for an actor to perform in and understand. That they can immerse themselves in and allows them to engage with their character totally.
Continue reading »
One is a beautiful amalgamation of fragility, poignancy, irreverence and razor-sharp wit. The other is a breathtaking journey through a Victorian metropolis that reflects on the inescapable pervasiveness of bigotry, prejudice, racism and intolerance. Meet the cinematographer behind “Fleabag” and “Carnival Row”.
Continuing the ongoing series of interviews with creative artists working on various aspects of movie and TV productions, it is my pleasure to welcome Tony Miller. In this interview he talks about the beginning of his career shooting documentaries, the transition of the industry from film to digital, various facets of the role of a cinematographer, and choosing his projects. Around these topics and more, Tony dives deep into his work on the first season of “Carnival Row” and two seasons of “Fleabag”.
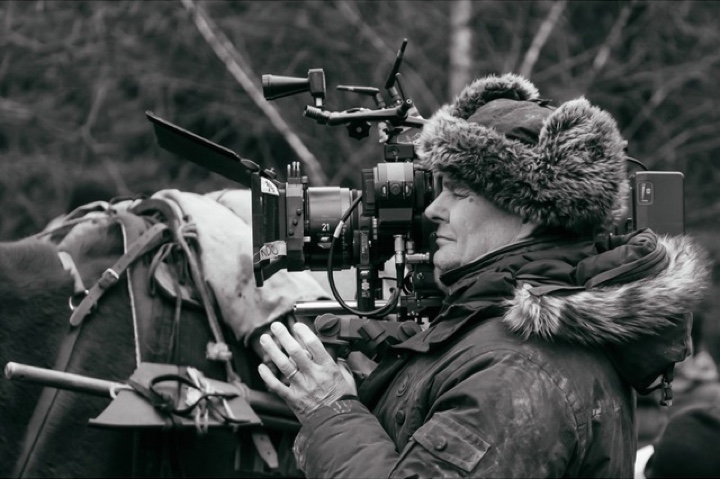
Kirill: Please tell us about yourself and the path that took you to where you are today.
Tony: I studied drama at Bristol university, as I thought that I wanted to be an actor but soon realised it was not my bag and I found myself drawn to cinematography.
When I left university, I self-funded a film documentary about the student uprising in Burma. I crossed the border illegally into Burma in 1988 at age 22 and filmed the first big genocide in which thousands of students were killed, and then crossed back with the film footage.
It was a big risk, but it helped kick-start my career, and I landed a big Channel 4 commission to go back and make a full one-hour documentary.
After the Burma experience, I spent 18 months filming a portrait of whale expert Dr Roger Payne. I then ended up shooting documentaries for the next 12 years, many were anthropological and that was an amazing time. There was a British tradition of verité documentary filmmaking and I was very lucky to join the tail end of it. I travelled with the same crew for about 9 months a year and was booked up often a year ahead. All was shot on film. By 26 I had been Emmy nominated and Emmy awarded.
I think that what it taught me was how to light with what I had. How to shoot handheld. What mattered and how to cut it in your head – work out what you needed to tell the story. You didn’t have a second chance or take. You really had to cover things very quickly and find the emotion and drama in how you shot those images. I found that it was a wonderful school for cinematography. All my heroes – Chris Menges, Roger Deakins, Robert Richardson had started in this way. (They all continue to insist on operating by the way).
Film was a craft and when you did not see it processed for some weeks you had to know exactly what you had shot. So, you shot tests – multiple tests when you had down time and worked out what latitude you had – what made things look more contrasty… What stock to use when the light was harsh.
Film was an expensive commodity, so you thought carefully about what you shot.
At some point I started shooting commercials, and then I started to be offered drama and fiction work. By my mid-30s, I realized that I didn’t want to be traveling around the world 9 months a year. I did my first big TV drama when I was 32, and my first movie a few years later. However, shooting fiction I soon realised that I was still often away 9 months a year!
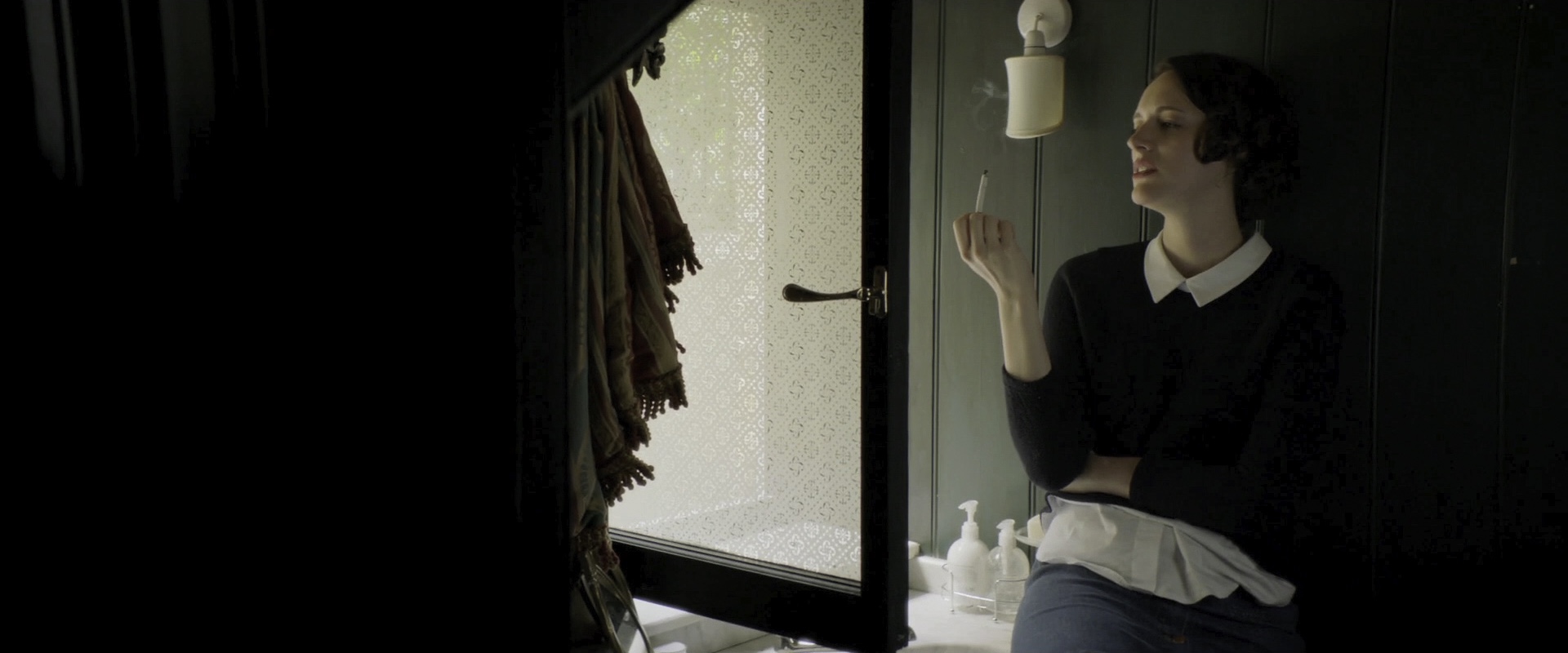
Cinematography of “Fleabag” by Tony Miller.
Kirill: Is there anything that still surprises you when you join a new production?
Tony: I’m always nervous the first few days, and I’m amazed how much there is still to learn. Every project is challenging, it is what makes it fascinating. I think if I felt it was easy, I wouldn’t do it anymore.
It’s still remarkably challenging on so many levels and the opportunity to do something new and different and sensitive is always there. Human nature is vast and so is underscoring the drama with light and a camera.
And on another level, you’re dealing with politics which is such a big part of our job. The bigger the production and the bigger the budget, the bigger the politics gets. Politics is a tricky business! You work with directors who are all very different and your relationship is always a close one. I’m continually surprised and fascinated by what we do.
Kirill: You mentioned that you started back in the days of film as medium, and nowadays digital is almost everywhere. Does it feel sometimes perhaps overwhelming how fast that technology is evolving in last 5-10 years?
Tony: Yes, it does. I’ve talked a lot at various events – BSC and Cameraimage about HDR and how it will take over all of our homes. I think it’s wonderful. I used to hate it, but after having seen “Roma” in HDR and also in a theatre, I thought that HDR looked more amazing and had more depth. I have now done six productions in that format and adapted to some degree how I shoot for HDR.
It is in a way a metaphor for how fast change comes at us these days. You have to keep up with the technology, but I have a crew that does some of those things for me.
But digital has opened the realm of cinematography from a niche profession that used to have maybe 30-40 DPs in Britain who were working at a high level, to now around 400-500. There are other reasons for it, such as people coming here from Europe and everybody seeming to want to shoot in England, but fundamentally it has made the craft of cinematography far more accessible and I think that is a great thing.
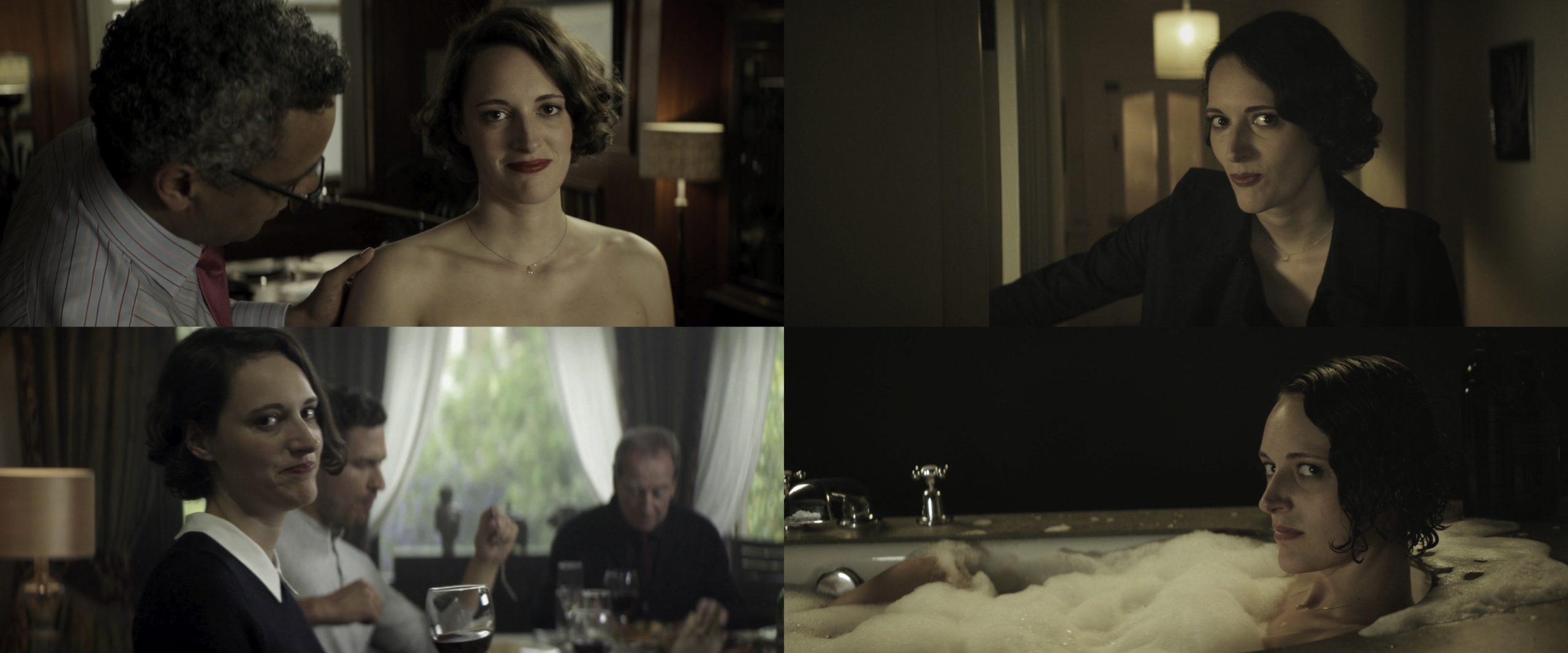
Breaking the fourth wall on “Fleabag”, cinematography by Tony Miller.
Kirill: How do you see the balance between the artistic side of what you do (finding the right way to tell that story) and the technological side of it (keeping track of all the latest cameras, gadgets and software)? Is one more important than the other for you?
Tony: But it’s all about the artistry. It’s all about how you read the story, how you tell the story, how you underscore the story, how you reveal the emotional beats. There are all the key elements that shape your approach on operating a camera and finding the decisive moment.
How do you crescendo to that moment? How do you back off? How do you underscore those key moments in a scene? Is it a close-up or is it a big wide shot? Experience really helps, but it doesn’t mean you’re always right. The big lesson in cinematography that I love is that often I’m right, but frequently I’m wrong.
Technology is really important. Those are our tools of the trade and they keep getting simpler and better – like LED lighting which is revolutionising how we work. I still use big tungsten sources, but LED is making me think and it saves time.
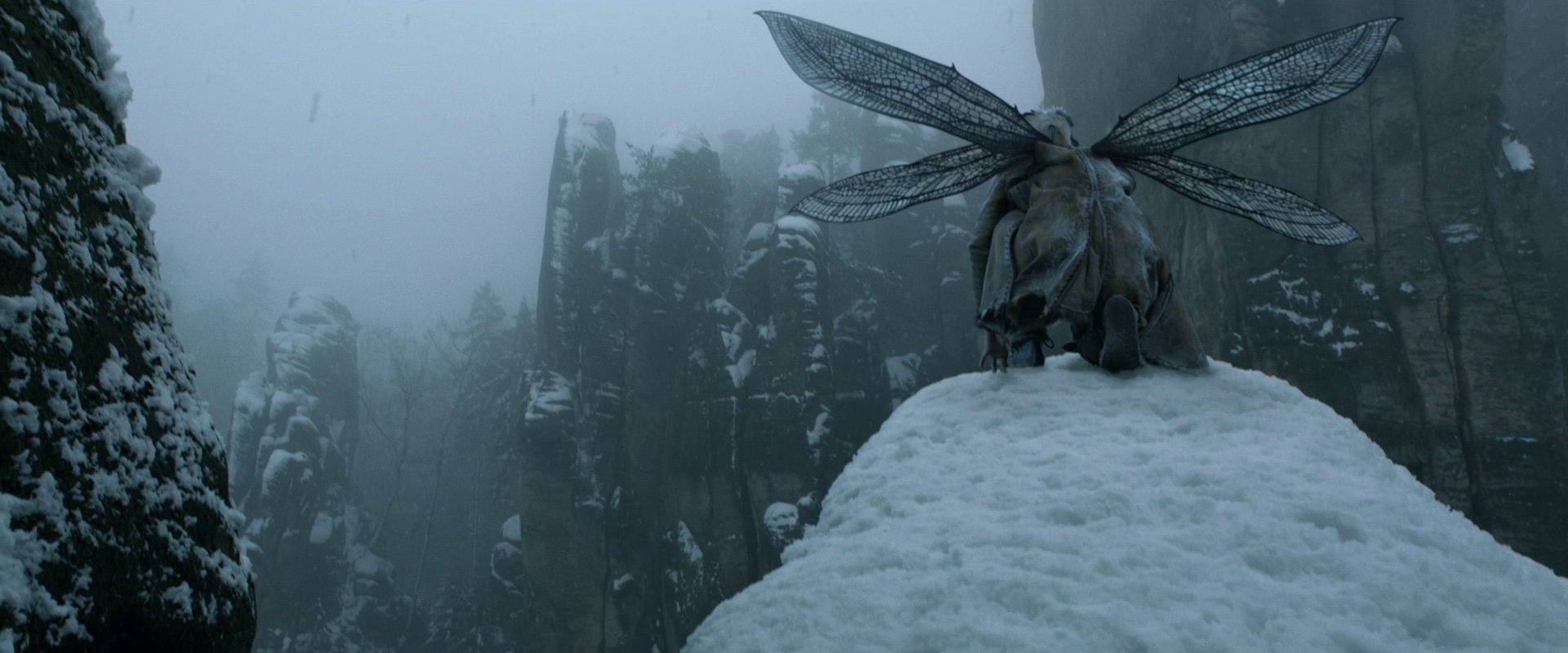
Cinematography of “Carnival Row” by Tony Miller.
Continue reading »
One last thing I wanted to do with hero images for interviews. Now on larger (desktop / laptop sized) screens the interview title blurb is displayed on top of a translucent scrim along the bottom edge of the hero image. Maybe now I’m done for 2019…
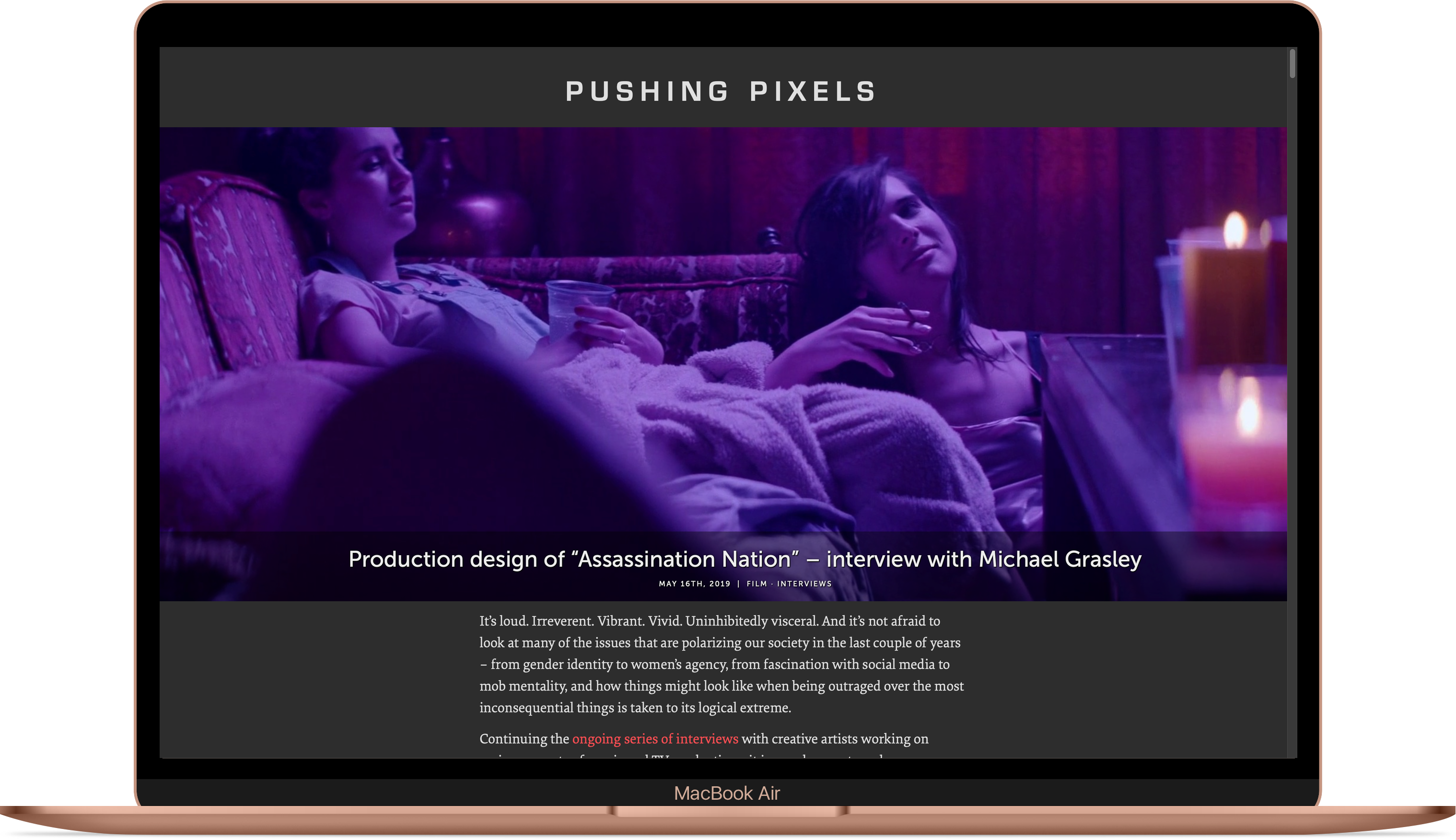
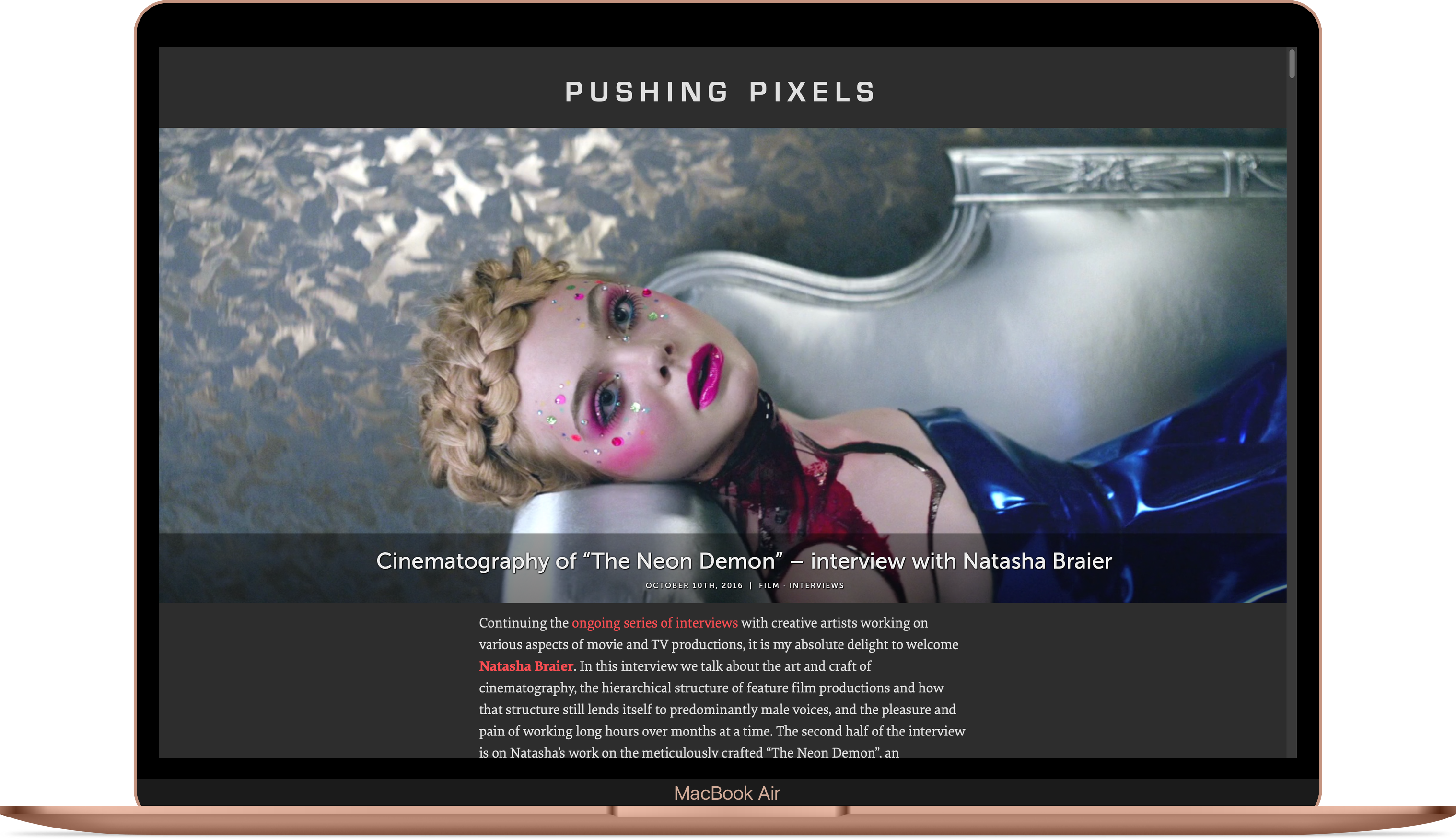